Hi
I am working on a project that require the OAK-D-PRO remain working 24/7.
I have tried to run the cam, but the cam seems to close itself after around 2.5 days. May I get some advices on what's the possible reason? and any suggestion on how to fix it?
Below is the debug log of the cam:
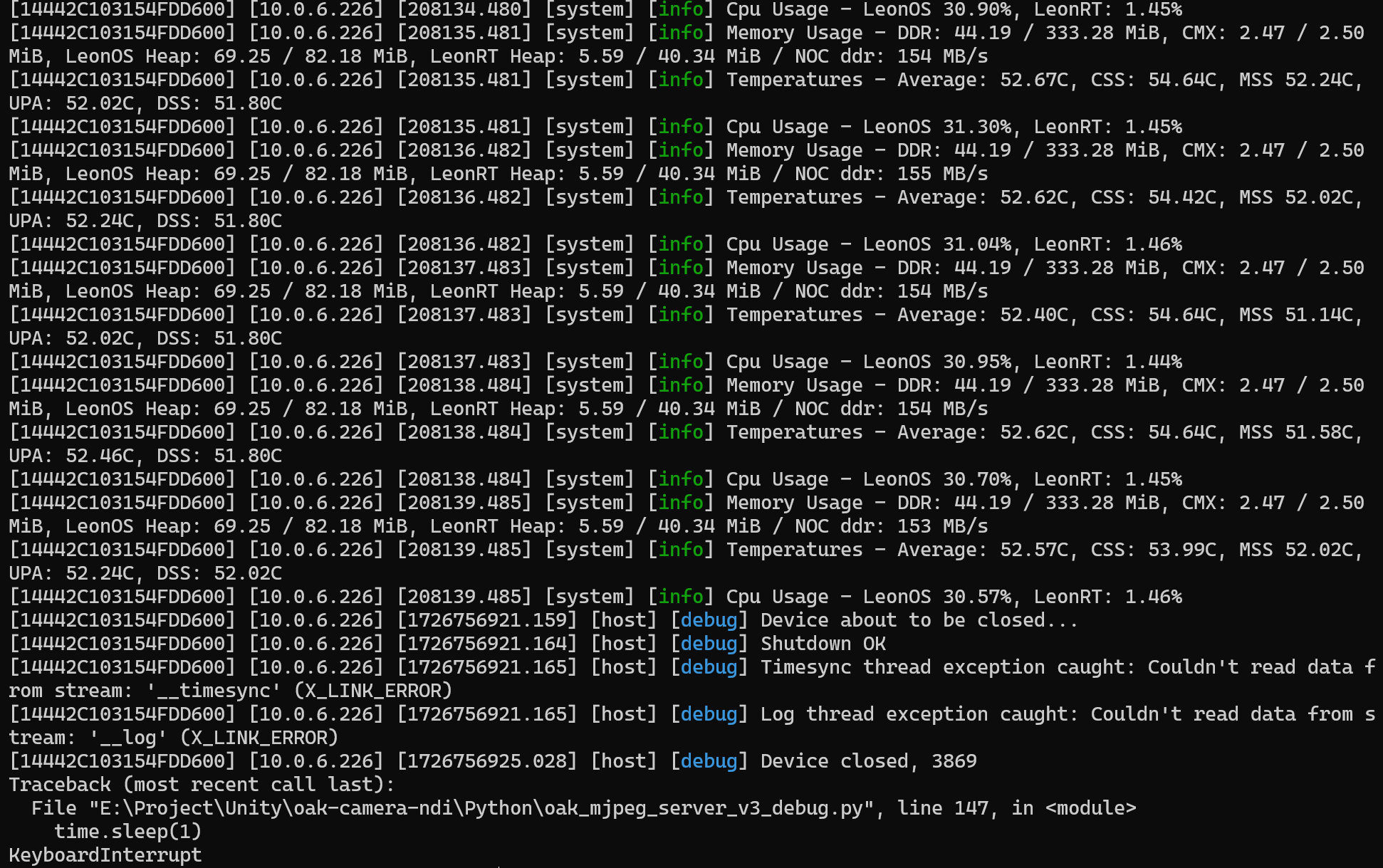
And the script I am running:
#!/usr/bin/env python3
import depthai as dai
import time
DesiredWidth=640
DesiredHeight=480
# Start defining a pipeline
pipeline = dai.Pipeline()
# Define a source - color camera
cam = pipeline.create(dai.node.ColorCamera)
# Create ImageManip node for resizing
manip = pipeline.create(dai.node.ImageManip)
manip.setMaxOutputFrameSize(DesiredWidth*DesiredHeight*3)
manip.initialConfig.setResize(DesiredWidth, DesiredHeight)
# VideoEncoder
color_jpeg = pipeline.create(dai.node.VideoEncoder)
color_jpeg.setDefaultProfilePreset(cam.getFps(), dai.VideoEncoderProperties.Profile.MJPEG)
# Define sources and outputs
monoLeft = pipeline.create(dai.node.MonoCamera)
monoRight = pipeline.create(dai.node.MonoCamera)
depth = pipeline.create(dai.node.StereoDepth)
# Properties
monoLeft.setResolution(dai.MonoCameraProperties.SensorResolution.THE_400_P)
monoLeft.setCamera("left")
monoRight.setResolution(dai.MonoCameraProperties.SensorResolution.THE_400_P)
monoRight.setCamera("right")
# Create a node that will produce the depth map (using disparity output as it's easier to visualize depth this way)
depth.setDefaultProfilePreset(dai.node.StereoDepth.PresetMode.HIGH_DENSITY)
# Options: MEDIAN_OFF, KERNEL_3x3, KERNEL_5x5, KERNEL_7x7 (default)
depth.initialConfig.setMedianFilter(dai.MedianFilter.KERNEL_7x7)
depth.setLeftRightCheck(True) # Better handling for occlusions
depth.setExtendedDisparity(False) # Closer-in minimum depth, disparity range is doubled (from 95 to 190)
depth.setSubpixel(False) # Better accuracy for longer distance, fractional disparity 32-levels
config = depth.initialConfig.get()
config.postProcessing.speckleFilter.enable = False
# config.postProcessing.speckleFilter.speckleRange = 50
# config.postProcessing.temporalFilter.enable = True
# config.postProcessing.spatialFilter.enable = True
# config.postProcessing.spatialFilter.holeFillingRadius = 2
# config.postProcessing.spatialFilter.numIterations = 1
config.postProcessing.thresholdFilter.minRange = 0
config.postProcessing.thresholdFilter.maxRange = 10000
# config.postProcessing.decimationFilter.decimationFactor = 1
depth.initialConfig.set(config)
# VideoEncoder
depth_jpeg = pipeline.create(dai.node.VideoEncoder)
depth_jpeg.setDefaultProfilePreset(25, dai.VideoEncoderProperties.Profile.MJPEG)
# Script node
script = pipeline.create(dai.node.Script)
script.setProcessor(dai.ProcessorType.LEON_CSS)
script.setScript("""
import socket
import fcntl
import struct
from socketserver import ThreadingMixIn
from http.server import BaseHTTPRequestHandler, HTTPServer
PORT = 8080
def get_ip_address(ifname):
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
return socket.inet_ntoa(fcntl.ioctl(
s.fileno(),
-1071617759, # SIOCGIFADDR
struct.pack('256s', ifname[:15].encode())
)[20:24])
class ThreadingSimpleServer(ThreadingMixIn, HTTPServer):
pass
class HTTPHandler(BaseHTTPRequestHandler):
def do_GET(self):
if self.path == '/':
self.send_response(200)
self.end_headers()
self.wfile.write(b'<h1>[DepthAI]</h1>')
self.wfile.write(b'<p>Click <a href="color">here</a> for color camera</p>')
self.wfile.write(b'<p>Click <a href="depth">here</a> for depth camera</p>')
elif self.path == '/color':
try:
self.send_response(200)
self.send_header('Content-type', 'multipart/x-mixed-replace; boundary=--jpgboundary')
self.end_headers()
while True:
jpegImage = node.io['color_jpeg'].get()
self.wfile.write("--jpgboundary".encode())
self.wfile.write(bytes([13, 10]))
self.send_header('Content-type', 'image/jpeg')
self.send_header('Content-length', str(len(jpegImage.getData())))
self.end_headers()
self.wfile.write(jpegImage.getData())
self.end_headers()
except Exception as ex:
node.warn(str(ex))
elif self.path == '/depth':
try:
self.send_response(200)
self.send_header('Content-type', 'multipart/x-mixed-replace; boundary=--jpgboundary')
self.end_headers()
while True:
jpegImage = node.io['depth_jpeg'].get()
self.wfile.write("--jpgboundary".encode())
self.wfile.write(bytes([13, 10]))
self.send_header('Content-type', 'image/jpeg')
self.send_header('Content-length', str(len(jpegImage.getData())))
self.end_headers()
self.wfile.write(jpegImage.getData())
self.end_headers()
except Exception as ex:
node.warn(str(ex))
with ThreadingSimpleServer(("", PORT), HTTPHandler) as httpd:
node.warn(f"Serving at {get_ip_address('re0')}:{PORT}")
httpd.serve_forever()
""")
# Connect camera to ImageManip and then to VideoEncoder
cam.video.link(manip.inputImage)
manip.out.link(color_jpeg.input)
color_jpeg.bitstream.link(script.inputs['color_jpeg'])
monoLeft.out.link(depth.left)
monoRight.out.link(depth.right)
depth.disparity.link(depth_jpeg.input)
depth_jpeg.bitstream.link(script.inputs['depth_jpeg'])
while True:
try:
# Connect to device with pipeline
with dai.Device(pipeline) as device:
while not device.isClosed():
time.sleep(1)
except Exception as ex:
print(ex)
time.sleep(5)
Any advices would be greatly appreciated.
Thanks in advance.