Hi @erik,
Just want to give you an update with the "zoom-in" issue. Change to the latest develop branch didn't make a difference. But it can be fixed by adding a scaling factor to the camera intrinsics array while creating the maps for undistortion.
Here is the updated function getMesh function for this example
def getMesh(calibData: dai.CalibrationHandler, ispSize, focal_len_scaling_factor: float = 1.0):
M1 = np.array(calibData.getCameraIntrinsics(camSocket, ispSize[0], ispSize[1]))
d1 = np.array(calibData.getDistortionCoefficients(camSocket))
R1 = np.identity(3)
M1_new = M1.copy()
M1_new[0][0] *= focal_len_scaling_factor
M1_new[1][1] *= focal_len_scaling_factor
mapX, mapY = cv2.initUndistortRectifyMap(M1, d1, R1, M1_new, ispSize, cv2.CV_32FC1)
meshCellSize = 16
mesh0 = []
# Creates subsampled mesh which will be loaded on to device to undistort the image
for y in range(mapX.shape[0] + 1): # iterating over height of the image
if y % meshCellSize == 0:
rowLeft = []
for x in range(mapX.shape[1]): # iterating over width of the image
if x % meshCellSize == 0:
if y == mapX.shape[0] and x == mapX.shape[1]:
rowLeft.append(mapX[y - 1, x - 1])
rowLeft.append(mapY[y - 1, x - 1])
elif y == mapX.shape[0]:
rowLeft.append(mapX[y - 1, x])
rowLeft.append(mapY[y - 1, x])
elif x == mapX.shape[1]:
rowLeft.append(mapX[y, x - 1])
rowLeft.append(mapY[y, x - 1])
else:
rowLeft.append(mapX[y, x])
rowLeft.append(mapY[y, x])
if (mapX.shape[1] % meshCellSize) % 2 != 0:
rowLeft.append(0)
rowLeft.append(0)
mesh0.append(rowLeft)
mesh0 = np.array(mesh0)
meshWidth = mesh0.shape[1] // 2
meshHeight = mesh0.shape[0]
mesh0.resize(meshWidth * meshHeight, 2)
mesh = list(map(tuple, mesh0))
return mesh, meshWidth, meshHeight
And here are the undistorted images with scaling factor=1.0, 0.8 and 0.6. Smaller the factor, the more "zoom out" the image is.
Thanks
Lincoln
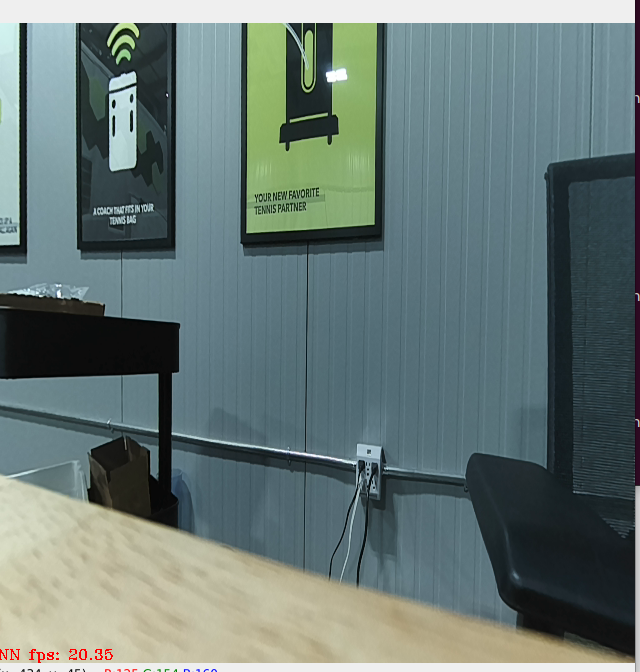
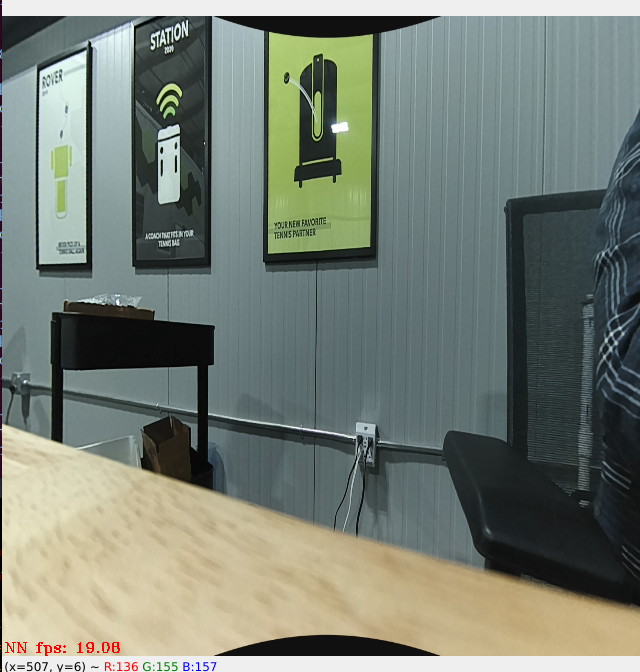
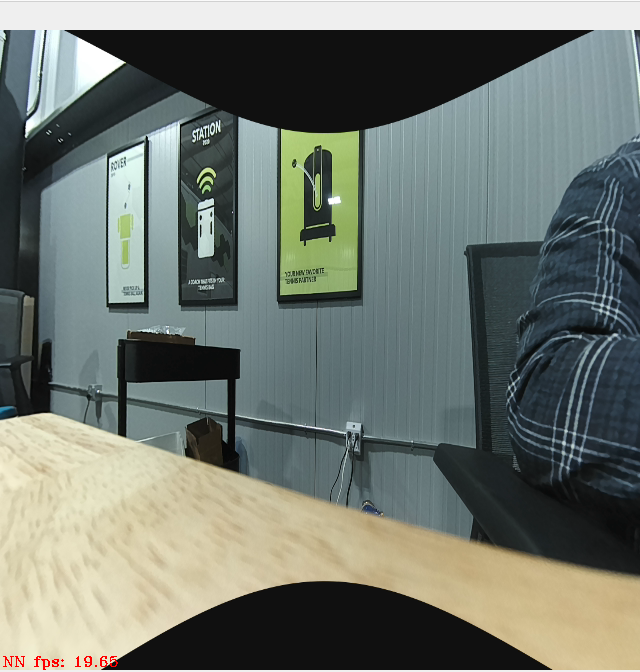