Dear all, it's nice to be in the community, thank you very much for setting up!
I recently bought an OAK D Lite and I'm trying to get warm with it. For my next task, I decided to just display the video of the sensors and take pictures with a Key-Press. I wanted to try the camera-node instead of the monocamera-node.
Unfortunately I'm getting really weird results with the outcome of the rgbcam. It's as if the inputs of both, the bw-sensors as well as the input of the rgb-cam is beeing used to create my outcome. You can see the result in the attachment. The output of the rightcam behaves as it should.
Here is the code I'm using:
import depthai as dai
import cv2
# Create pipeline
pipeline = dai.Pipeline()
# Define sources and outputs
camRgb = pipeline.create(dai.node.Camera)
camright = pipeline.create(dai.node.Camera)
camright.setCamera('right')
stillRGBEncoder = pipeline.create(dai.node.VideoEncoder)
stillBWREncoder = pipeline.create(dai.node.VideoEncoder)
controlIn = pipeline.create(dai.node.XLinkIn)
rControlIn = pipeline.create(dai.node.XLinkIn)
videoOut = pipeline.create(dai.node.XLinkOut)
stillMjpegOut = pipeline.create(dai.node.XLinkOut)
videoROut = pipeline.create(dai.node.XLinkOut)
stillBWRMjpegOut = pipeline.create(dai.node.XLinkOut)
controlIn.setStreamName('controlrgb')
rControlIn.setStreamName('rcontrol')
videoOut.setStreamName('videorgb')
videoROut.setStreamName('videoright')
stillMjpegOut.setStreamName('rgbstill')
stillBWRMjpegOut.setStreamName('rstill')
# Properties
camRgb.setVideoSize(640,360)
stillRGBEncoder.setDefaultProfilePreset(1, dai.VideoEncoderProperties.Profile.MJPEG)
stillBWREncoder.setDefaultProfilePreset(1, dai.VideoEncoderProperties.Profile.MJPEG)
# Linking
# RGB
camRgb.still.link(stillRGBEncoder.input)
camRgb.video.link(videoOut.input)
# Mono
camright.still.link(stillBWREncoder.input)
camright.video.link(videoROut.input)
# Control
controlIn.out.link(camRgb.inputControl)
rControlIn.out.link(camright.inputControl)
stillRGBEncoder.bitstream.link(stillMjpegOut.input)
stillBWREncoder.bitstream.link(stillBWRMjpegOut.input)
# Connect to device and start pipeline
with dai.Device(pipeline) as device:
# Get data queues
controlQueue = device.getInputQueue('controlrgb')
rControlQueue = device.getInputQueue('rcontrol')
videoQueue = device.getOutputQueue('videorgb', maxSize = 1, blocking = False)
rightVQueue = device.getOutputQueue('videoright', maxSize = 1, blocking = False)
stillQueue = device.getOutputQueue('rgbstill', maxSize = 1, blocking = False)
stillrQueue = device.getOutputQueue('rstill', maxSize = 1, blocking = False)
while True:
BWRFrames = rightVQueue.tryGetAll()
for BWRFrame in BWRFrames:
cv2.imshow('RightVideo', BWRFrame.getCvFrame())
vidFrames = videoQueue.tryGetAll()
for vidFrame in vidFrames:
cv2.imshow('video', vidFrame.getCvFrame())
stillbwrFrames = stillrQueue.tryGetAll()
for bwrframe in stillbwrFrames:
rframe = cv2.imdecode(bwrframe.getData(), cv2.IMREAD_UNCHANGED)
cv2.imshow('bwrstill', rframe)
stillrgbFrames = stillQueue.tryGetAll()
for stillFrame in stillrgbFrames:
# Decode JPEG
cframe = cv2.imdecode(stillFrame.getData(), cv2.IMREAD_UNCHANGED)
# Display
cv2.imshow('still', cframe)
# Update screen
key = cv2.waitKey(1)
if key == ord('q'):
break
elif key == ord('c'):
ctrl = dai.CameraControl()
ctrl.setCaptureStill(True)
controlQueue.send(ctrl)
rControlQueue.send(ctrl)
This is the result I'm getting with the rgb-cam
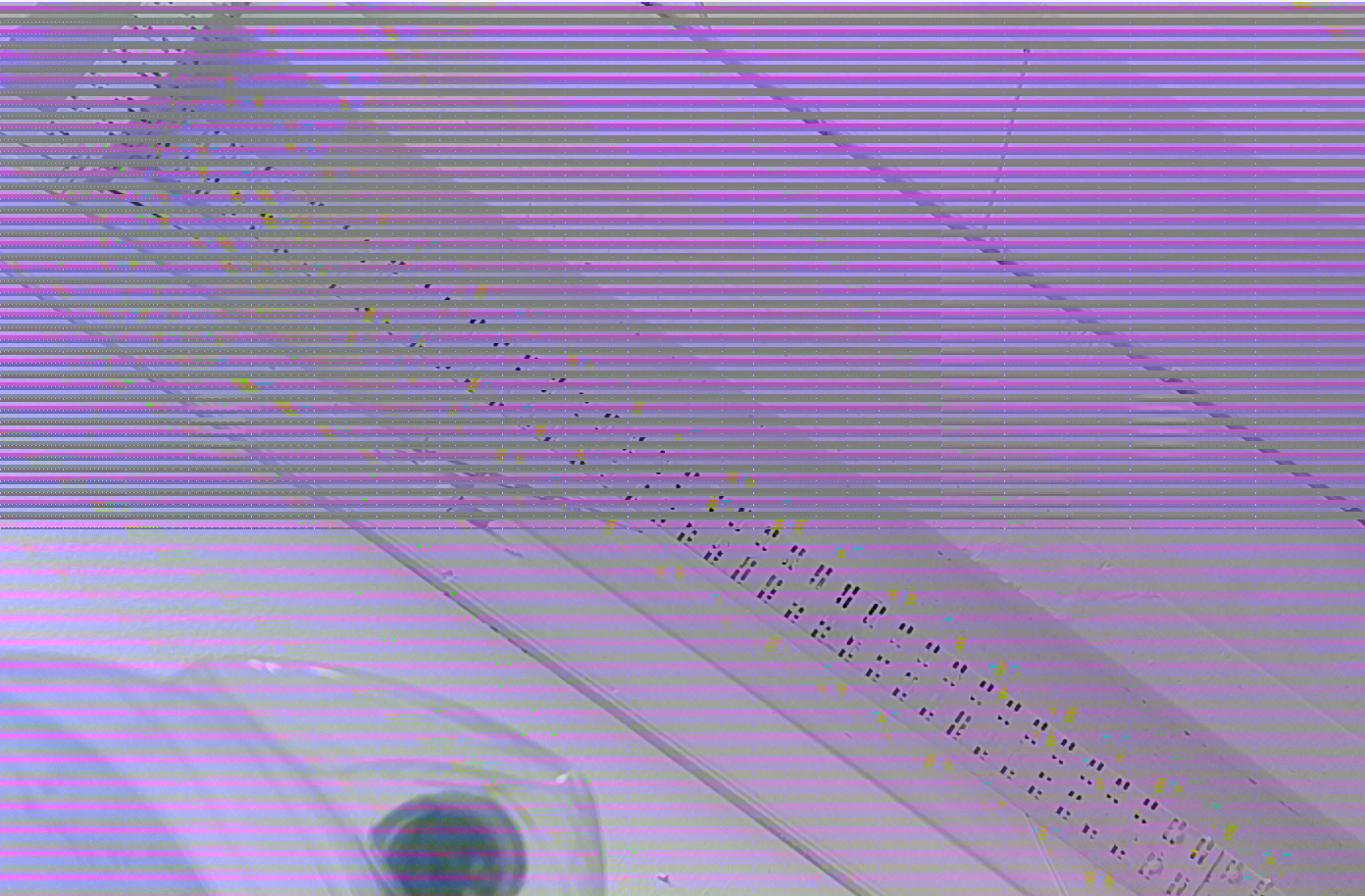
I already triple-checked if I messed up the connection of the modules but can't find any mistakes here.
I'd be very thankful if someone could help me out here. Maybe it has got something to do with the Camera-Node I'm using?
It's all working quite well, when I'm just using the monocamera-Node for the left and right sensor.